Introduction
Why would we clear the Git commits and history from the local & remote master repository? When preparing training labs leveraging Azure DevOps and Git, I often need to do a lot of testing and experimenting to empirically get the scenarios right. That means the commit history is cluttered with irrelevant commits for the lab training I am presenting.
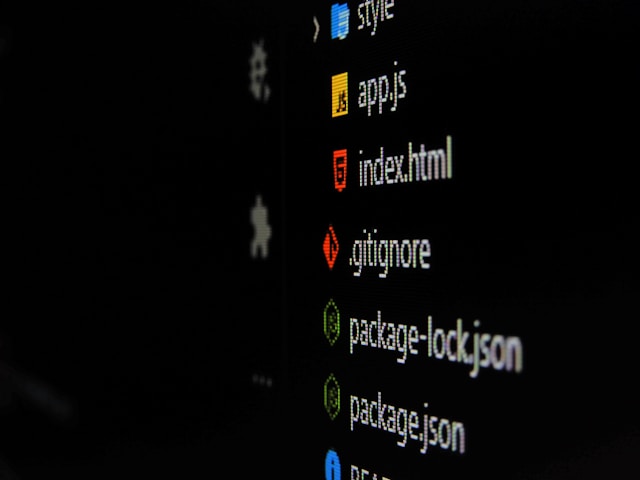
Ideally, I reset the history to start a training lab when the repository is at the right stage. The students are then not bothered by the commits of previous demos. But how can we clear the Git commits and history from the local & remote master repository?
Clear the Git commits and history from the local & remote master repository
Git is meant to keep the commit history, as are repositories like Azure DevOps. That means there is no way to reset the commit history in Azure DevOps. Git, being a very powerful and, to a certain extent, also a dangerous tool, can help you overcome this. But how to do it is not always obvious. That said, you can also shoot yourself in the foot with Git, so pay attention and be careful.
Step 1 to Clear the Git commits and history from the local & remote master repository
If you keep branches around things get complicated. For my needs, I don’t need them. To delete a branch via git we need three (3) deletes.
git push origin --delete marshipdev
git branch --delete marshipdev
git branch --delete --remotes origin/marshipdev
The above lines respectively delete:
You can also delete remote branches in Azure DevOps via the GUI by selecting a branch and selecting “Delete branch” in the menu. Locally you’ll need to use Git commands or the Git GUI.
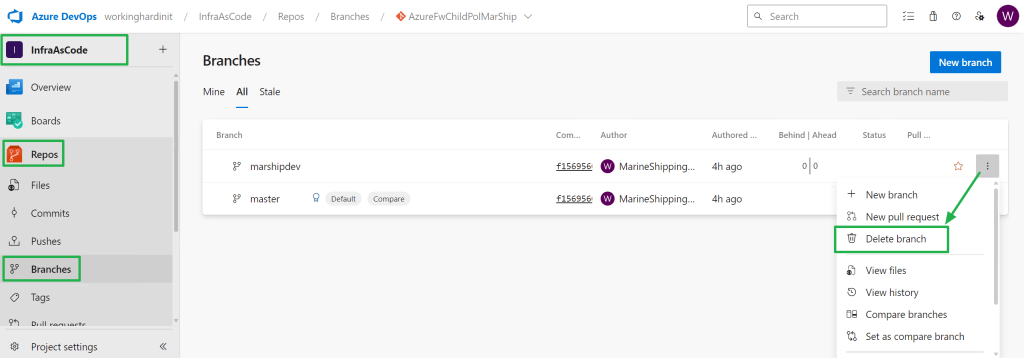
Step 2
Create a new orphaned branch
git checkout --orphan myresetbranch
The Git option –orphan creates a branch that is in a git init “like” state. That is why we have an alternative option and that is to delete the .git folder in your local repository and run git init in it. That is why i normally keep a copy around of the “perfect” situation with the .git folder removed. I can copy that to create a new local master branch by running git init. I then have that track a new remote repository that still needs initializing via:
git remote add origin https://[email protected]/workinghardinit/InfraAsCode/_git/AzureFwChildPolMarShip
git push --set-upstream origin master
But that is not what I am doing here, I am using another method.
Step 3
On your workstation in the local repository, make sure to clean and delete or edit and add all the files and folders we want to be in our master repository initial commit.
git add -A
git commit -m “Initial commit”
Note: we use -A here instead of “.” Because we also want to delete any tracked files and folder that are currently being tracked. At the same time, it adds new items to be tracked. In practice it is like running both git -u and git .
Step 4
Now delete the current master branch
git branch -D master
Step 5
Rename the temporary branch to “master”
git branch -m master
We now have a master repository again locally.
Step 6
We now need to update the remote repository with the option –force or -f. That allows us to delete branches and tags as well as rewrite the history. Normally that is no allowed so we nee to temporarily allow this in Azure DevOps.
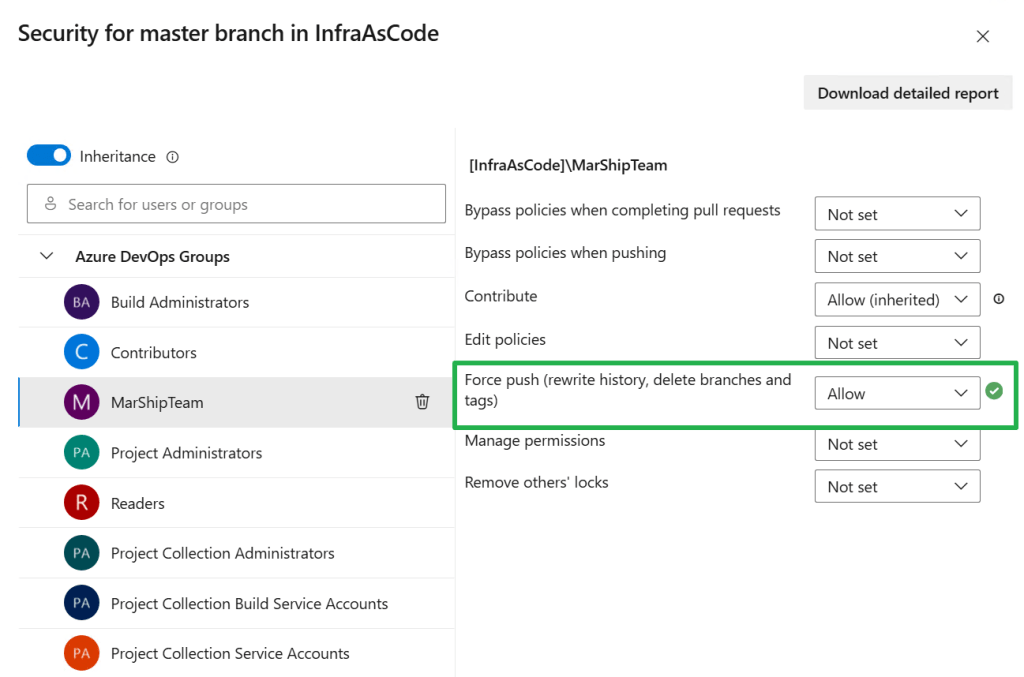
Now we can run
git push -f origin master
If we had not allowed Force push the above command would fail with an error indicating we need to allow “Force push”.
TF401027: You need the Git ‘ForcePush’ permission to perform this action.
Important: do not forget to set “Force push” back to “Not set”
Step 7 to Clear the Git commits and history from the local & remote master repository
Finally, make sure that the local master branch is set up to track origin/master.
git push --set-upstream origin master
That’s it, you now have a master repository in Azure DevOps that is ready to be cloned and used for labs with a clean commit history. Student can clone it, create branches, work on that repository and they will only see their changes and commit.
Conclusion
Resetting the git commit history of a repository is not a recommend action on production repositories under normal situations. But in situations like training lab repositories, it gives me a clean commit history to start my demos from.